Update MySQL using HTML Form and PHP
This post will show you how to create a sample form to Update User Data in mySQL database Table using PHP scripts
(1) 3 x PHP file will be created “infoEdit” “infoEditForm ” “infoUpdate”
(2) First PHP File-> infoEdit-> This PHP file will connect the Database, -> When Successful Connected it will Echo out the Number of User available in mySQL database table to be Updated
(3) Second PHP File->infoEditForm -> This PHP File will connect the Database -> Collect the User input via $_POST function in the HTML Form.
(4) Third PHP File->infoUpdate -> This PHP File will connect the Database -> Update the User Inputted data into mySQL database.
Prior to that you need to complete the items listed Below
- Mamp or xampp with phpMyAdmin install into your Computer , check out here
- A Sample Database created Check out Here
- New User Created . Check out Here
- A New table created , check out Here
- Insert Data into the Table Here
The “infoEdit ” PHP File
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 | <?php /* * File: infoEdit.php * * *===================================== */ $hostName = "localhost" ; $userName = "jane" ; $userPassword = "jane" ; $database = "janedb" ; $dbConnectionStatus = new mysqli( $hostName , $userName , $userPassword , $database ); //Connection Error if ( $dbConnectionStatus ->connect_error){ die ( "Connection failed: " . $dbConnectionStatus ->connect_error); } // Connected to Database JaneDB // Object oriented -> pointing if ( $dbConnectionStatus ->query( "SELECT DATABASE()" )){ $dbSuccess =true; // $result = $dbConnectionStatus ->query( "SELECT DATABASE()" ); $row = $result ->fetch_row(); printf( "Default database is %s.\n" , $row [0]); $result ->close(); } if ( $dbSuccess ) { $selectData = " SELECT * FROM testtable" ; // Send Select Query $selectData_Query = mysqli_query( $dbConnectionStatus , $selectData ); echo '<form action="infoEditForm.php" method="post">' ; echo '<select name="infoID">' ; echo '<option value="0" label="" selected="selected">..Select Name..</option>' ; if (mysqli_num_rows( $selectData_Query )>0){ // while there is still data in row // assign selected mySQL data into 'arrayData' array while ( $rows =mysqli_fetch_assoc( $selectData_Query )){ $ID = $rows [ 'id' ]; $companyName = $rows [ 'companyname' ]; $firstName = $rows [ 'firstname' ]; //Populate the Option echo '<option value="' . $ID . '">' . $firstName . '</option>' ; } } echo '</select>' ; echo '<input type="submit" />' ; echo '</form>' ; } echo "<br /><hr /><br />" ; echo '<a href="/">Back to LocalHost</a><br />' ; ?> |
The “infoEditForm ” PHP File
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 | <?php /* * File: infoEditForm.php * * *===================================== */ $hostName = "localhost" ; $userName = "jane" ; $userPassword = "jane" ; $database = "janedb" ; $dbConnectionStatus = new mysqli( $hostName , $userName , $userPassword , $database ); //Connection Error if ( $dbConnectionStatus ->connect_error){ die ( "Connection failed: " . $dbConnectionStatus ->connect_error); } // Connected to Database JaneDB // Object oriented -> pointing if ( $dbConnectionStatus ->query( "SELECT DATABASE()" )){ $dbSuccess =true; // $result = $dbConnectionStatus ->query( "SELECT DATABASE()" ); $row = $result ->fetch_row(); printf( "Default database is %s.\n" , $row [0]); $result ->close(); } if ( $dbSuccess ) { // Get the details of the company selected $infoID = $_POST [ "infoID" ]; if ( $infoID ==0){ // If nothing is selected header( 'Location:infoEdit.php' ); } // Select Query base on Info Id $cname__select = "SELECT * FROM testtable WHERE id = $infoID" ; // Execute Query $cname__select_Query = mysqli_query( $dbConnectionStatus , $cname__select ); // While there is info in row while ( $rows =mysqli_fetch_assoc( $cname__select_Query )){ $Id = $rows [ 'id' ]; $firstName = $rows [ 'firstname' ]; $lastName = $rows [ 'lastname' ]; $emails = $rows [ 'email' ]; $companyName = $rows [ 'companyname' ]; } } echo '<h2 style= "font-family: arial, helvetica, sans-serif;" > User Information Update Form </h2>'; // FORM postInfo User echo '<form name="postCompany" action="infoUpdate.php" method="post">' ; echo '<input type="hidden" name="infoID" value="' . $Id . '"/>' ; echo ' <table> <tr> <td>First Name</td> <td><input type= "text" name= "firstName" value= "'.$firstName.'" /></td> </tr> <tr> <td>Last Name</td> <td><input type= "text" name= "lastName" value= "'.$lastName.'" /></td> </tr> <tr> <td>Email</td> <td><input type= "text" name= "emails" value= "'.$emails.'" /></td> </tr> <tr> <td>Company</td> <td><input type= "text" name= "companyName" value= "'.$companyName.'" /></td> </tr> <tr> <td></td> <td align= "right" ><input type= "submit" value= "Save" /></td> </tr> </table> '; echo '</form>' ; echo "<br /><hr /><br />" ; echo '<a href="infoEdit.php">Select a different company</a>' ; echo " " ; echo '<a href="../index.php">Quit - to homepage</a>' ?> |
The “infoUpdate ” PHP File
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | <?php $hostName = "localhost" ; $userName = "jane" ; $userPassword = "jane" ; $database = "janedb" ; $dbConnectionStatus = new mysqli( $hostName , $userName , $userPassword , $database ); //Connection Error if ( $dbConnectionStatus ->connect_error){ die ( "Connection failed: " . $dbConnectionStatus ->connect_error); } // Connected to Database JaneDB // Object oriented -> pointing if ( $dbConnectionStatus ->query( "SELECT DATABASE()" )){ $dbSuccess =true; // $result = $dbConnectionStatus ->query( "SELECT DATABASE()" ); $row = $result ->fetch_row(); printf( "Default database is %s.\n" , $row [0]); $result ->close(); } if ( $dbSuccess ) { //collect the data with $_POST $ID = $_POST [ "infoID" ]; $firstName = $_POST [ "firstName" ]; $lastName = $_POST [ "lastName" ]; $companyName = $_POST [ "companyName" ]; $emails = $_POST [ "emails" ]; $companyName = $_POST [ "companyName" ]; // SQL Query $cname__select = "UPDATE testtable SET firstname = '$firstName' , lastname= '$lastName' , email ='$emails' ,companyname ='$companyName' WHERE id = $ID " ; //Execute Query $cname__select_Query = mysqli_query( $dbConnectionStatus , $cname__select ); if ( empty ( $firstName )) { echo '<span style="color:red; ">You Must Select First Name</span><br /><br />' ; } else { if ( $cname__select_Query ) { echo 'used to Successfully update Info.<br /><br />' ; } else { echo '<span style="color:red; ">FAILED to update Info.</span><br /><br />' ; } } } echo "<br /><hr /><br />" ; echo '<a href="infoEdit.php">Update another User</a>' ; echo " " ; echo '<a href="/">Back to LocalHost</a><br />' ; ?> |
The “infoEditForm ” PHP File
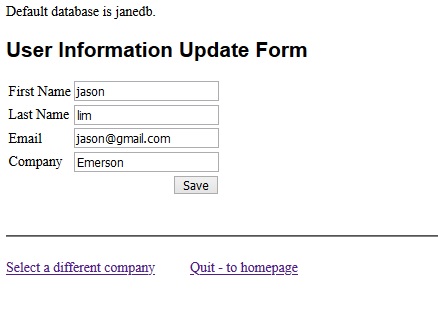
The “infoUpdate ” PHP File
mySQL