Java Switch Statement
Java Switch Statement.The conditional statement provide programmer the ability to step through different cases under different condition. For instance when a switch is trigger there might be different cases or condition within the switch .
Each single different condition within a switch , is called a case. When the Case is being triggered,that specific code within the case will be executed.
In this article,i will code a sample program to ask the User if they want to buy a drink.If the answer is yes , the program will out put the variety of drinks which they can choose from. Subsequently the User choose their drinks and the system will output the price.
Let Step into the Code and see How to implement Java Switch Statement in the Sample Program below
All fire Up,lets code some program!
In the program below , i will create 2 methods to process the information from the user. The First Method will print out the selection of drinks which the User would be able to choose from. The Second Method is where the switch statement come in,this will process the input selection from the user and then select the specific case and output the data.In this Program i will skip the exception handling function when the user input the wrong data.
- Lets Create our first method , I named it “printMenu()”
- Print out all the Selection drinks which the user are able to choose from
- Now lets create our second function called public static void selectTea(int selectDrink )
- Create an int variable called “int selectDrink”inside the parentheses , we need to pass in the parameter later to switch the desire case that the user selected
- Create 3 Cases and a default cases in the Switch Statement
- Under each Case print out the drinks that the user select , the price, and say thank you to the Customer
- Lets go back to our main program , create 2 global variable “yesno” & ” inputOpton” we need to assign the input value from the user into this 2 variable later in our program.
- Create 2 new Scanner object call “inputScan1 and inputScan2” to take in the User Input
- Be nice, Print out a welcome statement to greet the Customer, and ask them whether they would like to buy a drink ?
- Grab the user input via the scanner object and assign the value into the “yesno”variable
- If the User Answer equal “y” call the printMenu() method
- Ask the User to input the Selection via the Scanner Object and pass the value into the inputOption Variable
- Now your user have selected the type of tea they want , called the “selectTea( )” method . and pass the user input inputOption into the method
- Way the go , lets celebrate we have done our first switch statement in Java
package oop2; import java.util.*; public class Bubbletea { static String yesno; static int inputOption; public static void main(String[] args) { Scanner inputScan1= new Scanner(System.in); Scanner inputScan2 = new Scanner(System.in); System.out.println("Hello Welcome do your want a Drink Today (y/n) ?"); yesno = inputScan1.nextLine(); if(yesno.contentEquals("y")){ printMenu(); inputOption =inputScan2.nextInt(); selectTea(inputOption); } else{ System.out.println("Come Again :-)a"); } } public static void printMenu(){ System.out.println("(1).Bubble Tea with milk"); System.out.println("(2).Macha Green Tea with milk"); System.out.println("(3).Yuzu Green Tea with milk"); System.out.println("Select your Option (1, 2 ,3)"); } public static void selectTea(int selectDrink ){ switch (selectDrink ) { case 1: System.out.println("You have selected Bubble Tea with milk"); System.out.println("That will be S$4.00"); System.out.println("Thank you and come again"); break; case 2: System.out.println("You have selected Green Tea with milk"); System.out.println("That will be S$4.50"); System.out.println("Thank you and come again"); break; case 3: System.out.println("You have selected Yuzu Green Tea with milk"); System.out.println("That will be S$4.80"); System.out.println("Thank you and come again"); break; default: System.out.println("Please Select the Correct Option"); break; } } }
The Output with Java Switch Statement incorporated should look as below
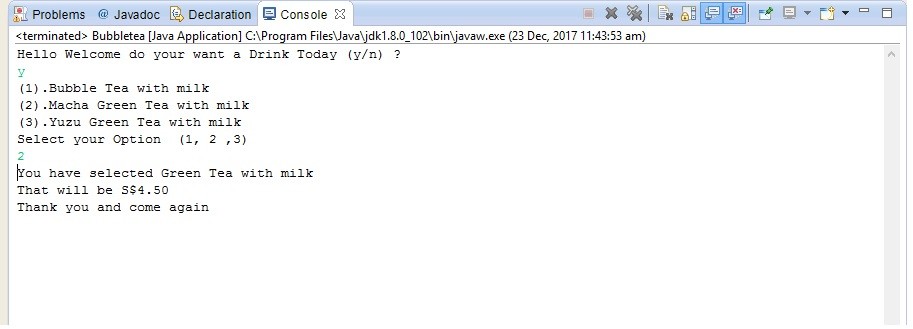
If you have any problem, you could always check out oracle website
Learn about if else conditional loop in Java here